How to create a Virtual Assistant AI Powered Bot such as Siri
Example of GPT BOT (Tressa)
Creating an end-to-end application to fine-tune a GPT model in Node.js and e
Creating an end-to-end application to fine-tune a GPT model in Node.js, expose it via an API, and consume that API from a React app with speech synthesis and voice recognition involves multiple steps. Below, I outline the general process you could follow:
Steps Overview
- Fine-tune the GPT model using Node.js and TensorFlow.js (or simply load a pretrained model).
- Create an API using Node.js with Express to serve the model.
- Create a React frontend to interact with the API and obtain model predictions.
- Implement speech synthesis and voice recognition in the React app.
Step 1: Fine-Tune the GPT Model with Node.js (Optional)
Please refer to the previous example to load or fine-tune a GPT model using TensorFlow.js and Node.js.
Step 2: Create an API Using Node.js and Express
// server.js
const express = require('express');
const bodyParser = require('body-parser');
const tf = require('@tensorflow/tfjs-node');
const app = express();
app.use(bodyParser.json());
async function loadModel() {
// Load or fine-tune your model here
}
const model = loadModel();
app.post('/predict', async (req, res) => {
const inputText = req.body.text;
// Perform inference with the model (simplified)
const prediction = 'Sample output for ' + inputText;
res.json({ prediction });
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000/');
});
Step 3: Create a React Frontend
Create a React app and include the necessary functionalities for speech synthesis and voice recognition.
// src/App.js in React app
import React, { useState } from 'react';
import axios from 'axios';
function App() {
const [text, setText] = useState('');
const [prediction, setPrediction] = useState('');
const getPrediction = async () => {
try {
const response = await axios.post('http://localhost:3000/predict', { text });
setPrediction(response.data.prediction);
// Perform speech synthesis
const speech = new SpeechSynthesisUtterance(response.data.prediction);
window.speechSynthesis.speak(speech);
} catch (error) {
console.error('There was an error making the prediction:', error);
}
};
const handleVoiceRecognition = () => {
const recognition = new (window.SpeechRecognition || window.webkitSpeechRecognition || window.mozSpeechRecognition || window.msSpeechRecognition)();
recognition.onresult = (event) => {
const transcript = event.results[0][0].transcript;
setText(transcript);
getPrediction();
};
recognition.start();
};
return (
<div className="App">
<button onClick={handleVoiceRecognition}>Start Voice Recognition</button>
<input type="text" value={text} onChange={(e) => setText(e.target.value)} />
<button onClick={getPrediction}>Predict</button>
<div>Prediction: {prediction}</div>
</div>
);
}
export default App;
Step 4: Enable CORS in Node.js (Optional)
To avoid CORS issues, you may need to enable CORS in your Node.js API:
Install CORS package:
npm install cors
Update server.js
:
const cors = require('cors');
app.use(cors());
Result:
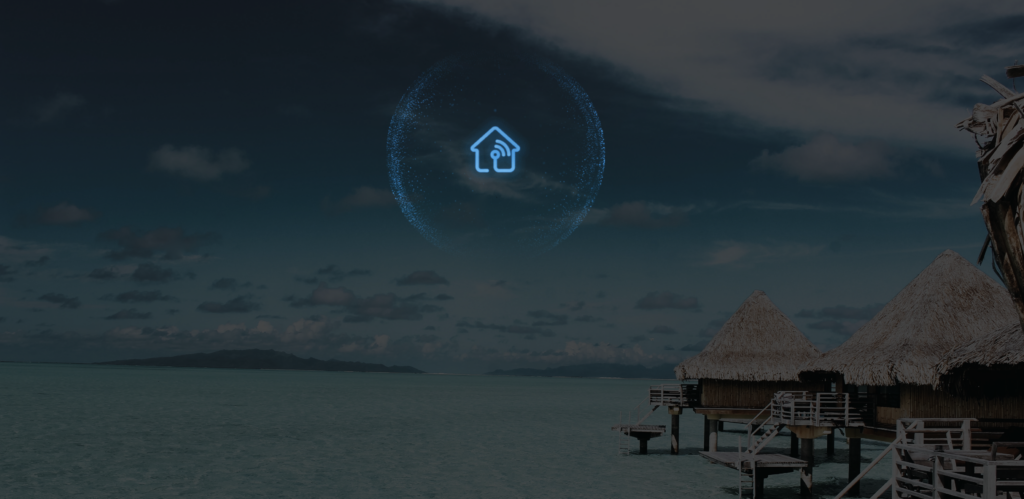
Useful Resources
GPT Models
- Introduction to GPT-2
- The Illustrated Transformer
- GPT-2 Simple Library for Fine-tuning
- GPT-3 API Documentation
- GPT-2 Language Models Tutorial
Node.js
- Node.js Official Website
- Express.js Documentation
- Getting Started with Node.js and TensorFlow.js
- Building a RESTful API using Node.js and Express
React
- React Official Website
- Create React App
- React Router for Single Page Applications
- State and Lifecycle in React
How to create a Virtual Assistant AI Powered Bot such as Siri