Javascript comes with built in features and methods that can be useful for solving complex problems.
In order to find a key of an Object in Javascript we can use following ES6 functions
The question of the day is we want to find the List of fields defined as keys in the following snippet of code.
const parkingLot = {
id: 1,
parkingLotName: 'A1',
costPerHours: 10,
reserved : true,
date: '27/12/2012'
}
The keys for parkingLot objects are :
- id
- parkingLotName
- costPerHours
- reserved
- date
The approach to the finding the list of allKeys would be
- Call the
Object.keys()
method to get an array of the object’s keys. - Using the
Object.hasOwnProperty ()
method to search for an individual key.
1.Using Object.keys()
approach to find list of keys in parkingLot object
const parkingLot = {
id: 1,
parkingLotName: "A1",
costPerHours: 10,
reserved: true,
date: "27/12/2012"
};
const Key = "parkingLotName";
const listOfAllKeys =
Object.keys(parkingLot);
// this will return ['id',
//'parkingLotName', 'costPerHours',
//'reserved', 'date']
const findKeyInArray =
listOfAllKeys.filter((currentKey)
=> currentKey === Key);
console.log({ findKeyInArray });
When you run the code the compiler will give the following output
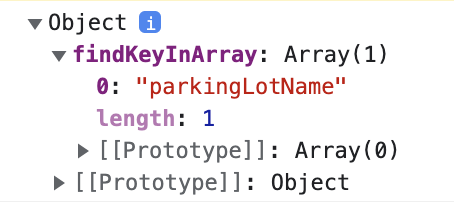
2. Using the Object.hasOwnProperty ()
method to search for an individual key.
javascript has also another Object.hasOwnProperty() method that we can use to search for an object key of an object
const parkingLot = {
id: 1,
parkingLotName: "A1",
costPerHours: 10,
reserved: true,
date: "27/12/2012"
};
const key = "parkingLotName";
const checkIfObjectHasId
= parkingLot.hasOwnProperty(key);
// this will return boolean
if (checkIfObjectHasId) {
console.log(
{ valueOfElement: parkingLot[key] }
);
// this will return 'A1'
}
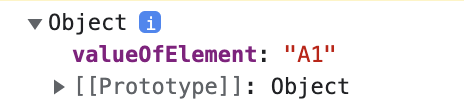
Learn Javascript using javascript book found on Amazon kindle
How to find keys of an object using javascript